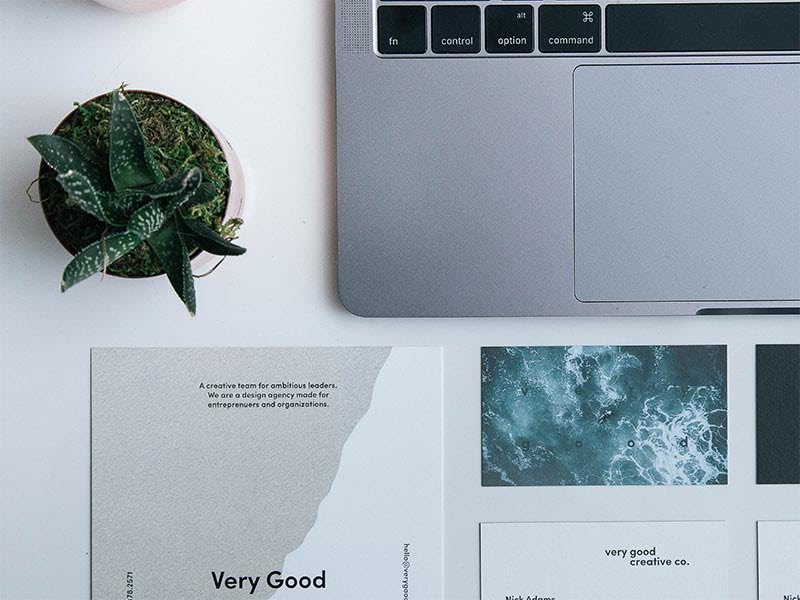
Transitioning a front-end project from development to production can be complex and challenging. Ensuring code quality and smooth deployment requires the right strategies and tools. In this post, I’ll share the coding guidelines and best practices I’ve implemented with my software development team to maintain high-quality, bug-free code. Whether you’re working individually or in a team, these best practices will help you optimize your workflow, boost efficiency, and improve project scalability.
Hi! I’m Reachea, and today I’m sharing my preferred coding guidelines and best practices, drawn from real-world experience, to ensure your code is both clean and maintainable.
Design a Clear Architecture and Folder Structure
One of the most crucial steps in any development process is establishing a clear project architecture and folder structure. Without it, your project can quickly become unmanageable as it grows.
Why Project Architecture Matters:
- Maintainability: A well-organized structure makes your codebase easy to navigate, simplifying updates, bug fixes, and collaboration.
- Scalability: A solid architecture ensures the project can grow without the need for major refactoring or starting over when adding new features.
- Efficient Refactoring: Grouping related components by domain helps developers make changes more efficiently, minimizing the time spent searching through the file tree.
Best Practices for Organizing Your Code:
- Organize by Domain: Group related files (components, services, utilities) by functionality to maintain a clean structure.
- Consistent Folder Hierarchy: Use clear, descriptive folder names and adhere to conventions that reflect the project’s structure and purpose.
Example: Code Without Architecture
Here’s an example of disorganized code with no clear architecture or separation of concerns:
/task-manager
index.html
app.js
styles.css
tasks.js
users.js
utils.js
services.js
components.js
Problems:
- All functionalities are crammed into a single directory.
- Difficult to maintain or scale the project.
- Testing and refactoring individual features is cumbersome.
Example: Code With Well-Defined Architecture
By comparison, this structured codebase adheres to best practices and is easier to maintain and extend.
File Structure:
/task-manager
/src
/components
TaskList.js
LoginForm.js
/services
taskService.js
authService.js
/utils
dateUtils.js
/styles
styles.css
index.js
index.html
With this architecture, responsibilities are well-separated, making the code more readable and scalable. Components can be reused and tested in isolation, reducing the likelihood of bugs.
Apply SOLID Principles
The SOLID principles are a set of object-oriented design guidelines that ensure your code is modular, maintainable, and scalable. Following these principles will help you avoid common pitfalls, such as tight coupling and inflexibility.The SOLID Principles:
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules but on abstractions.
- Single Responsibility Principle (SRP): A class should have one and only one reason to change.
- Open/Closed Principle (OCP): Classes should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subtypes should be substitutable for their base types.
- Interface Segregation Principle (ISP): No client should be forced to depend on methods it does not use.
Example: Code Without SOLID Principles
Without SOLID principles, the code quickly becomes rigid and difficult to maintain or scale:
class NotificationManager {
constructor() {
this.emailService = new EmailService();
this.smsService = new SmsService();
}
sendEmailNotification(user, message) {
this.emailService.sendEmail(user.email, message);
}
sendSmsNotification(user, message) {
this.smsService.sendSms(user.phoneNumber, message);
}
}
Problems:
- Tight coupling:
NotificationManager
depends directly onEmailService
andSmsService
. Any changes require modifyingNotificationManager
. - Violation of SRP and OCP: The class is responsible for multiple tasks (sending both email and SMS notifications), making it hard to extend.
Example: Code With SOLID Principles
By applying SOLID principles, the code becomes more modular and extensible:
// Abstraction for notification services
class NotificationService {
sendNotification(user, message) {
throw new Error('Method must be implemented');
}
}
class EmailNotificationService extends NotificationService {
sendNotification(user, message) {
console.log(`Sending email to ${user.email}: ${message}`);
}
}
class SmsNotificationService extends NotificationService {
sendNotification(user, message) {
console.log(`Sending SMS to ${user.phoneNumber}: ${message}`);
}
}
class NotificationManager {
constructor(notificationService) {
this.notificationService = notificationService;
}
sendNotification(user, message) {
this.notificationService.sendNotification(user, message);
}
}
Benefits:
- Loose coupling:
NotificationManager
depends on abstractions (NotificationService
), allowing it to be easily extended with new notification methods (e.g., push notifications) without modifying existing code. - Adheres to OCP: New notification types can be added by extending
NotificationService
without changingNotificationManager
.
Conclusion
By implementing clear architecture, following coding guidelines, and adhering to SOLID principles, you can dramatically improve your code’s quality, maintainability, and scalability. Whether you’re building a small project or working in a large team, these practices will help you write cleaner, more efficient code that’s easier to test and maintain over time.
Start applying these principles today, and watch your development process become smoother and more productive!