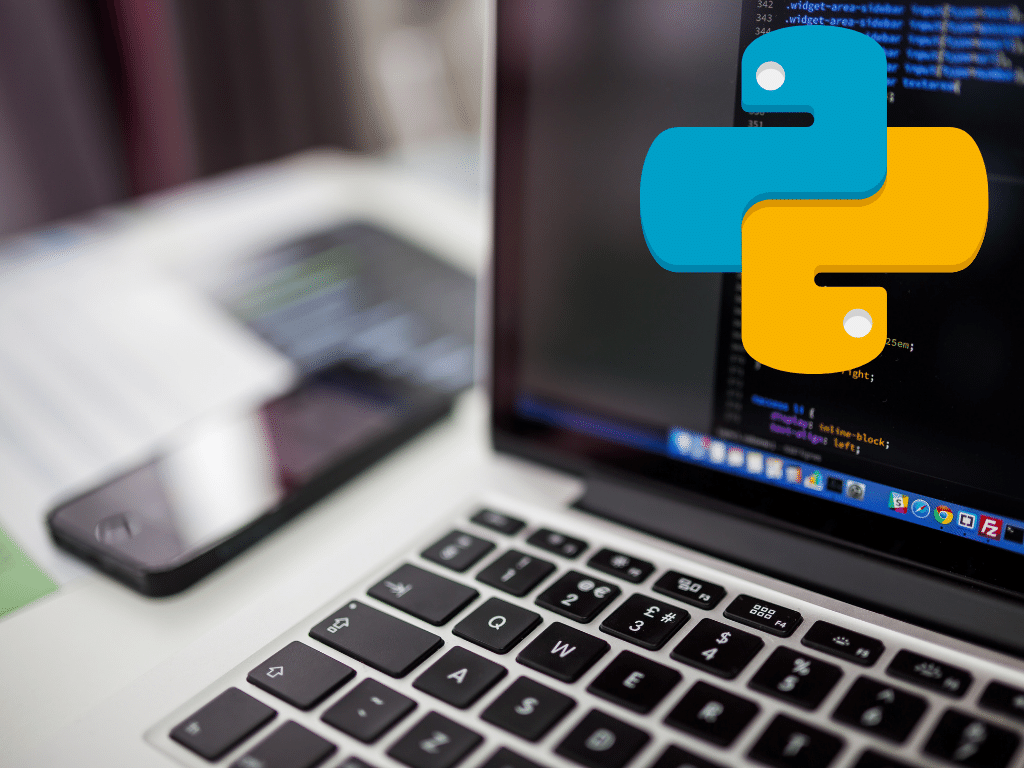
What is Programming?
Programming, derived from the Greek words “pro” (before) and “graphein” (to write), refers to the process of writing instructions in advance for computers to follow. At its core, programming is about creating solutions through logic and automation.
What is Python?
Python is one of the most popular programming languages today, known for its simplicity and versatility. With Python, you can instruct a computer to perform tasks automatically, from simple calculations to complex data processing. Python’s readability makes it an excellent choice for both beginners and seasoned developers.
Input and Output
Before diving into Python programming, it’s crucial to understand the concepts of input and output, as they form the backbone of how computer programs interact with users. A useful program must accept inputs and provide outputs.
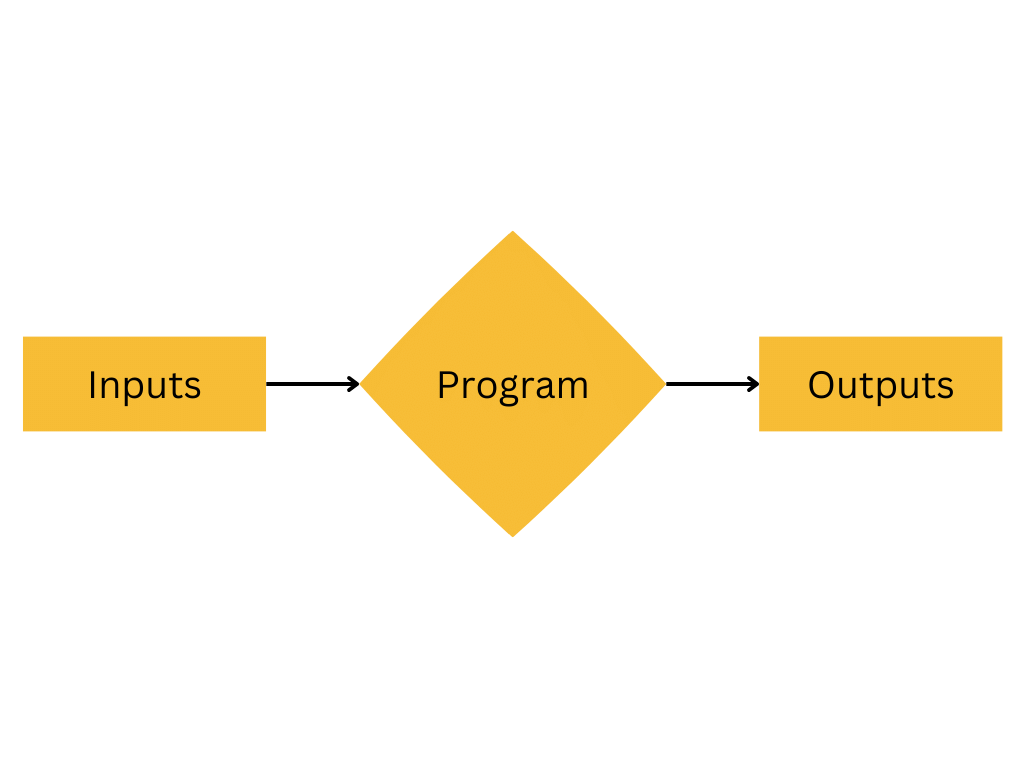
Common Input Methods:
- Touching: Through touchscreens.
- Clicking: Using the mouse.
- Typing: Via the keyboard.
Example of Output:
When you click on a browser icon, the computer responds by opening a new window—this is an example of graphical output. Outputs are how a computer responds to the inputs it receives.
Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) is software that helps you write, test, and debug your code. Choosing the right IDE for Python development is essential for efficiency. Some popular Python IDEs include:
- PyCharm
- VS Code
- Jupyter Notebooks
Each IDE offers unique features to streamline the coding process, from code autocompletion to built-in debugging tools.
Getting Started with Python: Printing Output
The simplest form of output in Python is text-based output, which you can produce using the print()
function. Here’s an example:
print('Hello, World!')
This will display Hello, World!
on the screen. In Python, text enclosed in quotes is known as a string. Understanding strings is fundamental, as they are one of the most common data types in Python.
Printing Multiple Lines
To avoid repetitive print()
statements, you can use special characters or multi-line strings. For example:
print("the\nbig\nblack\nbox")
The \n
character creates a new line for each word. Alternatively, Python allows multi-line strings using triple quotes:
print('''the
big
black
box''')
This approach is particularly useful when printing large blocks of text.
Variables and Concatenation
Variables allow you to store values that can be reused or changed throughout your program. For instance, consider this dialogue:
print('John: Hi Luke, long time no see.')
print('Luke: Hi John! How have you been?')
If you need to replace the name “John” with “James” throughout the script, changing every instance manually can be tedious. Instead, using variables can simplify this:
character_1 = 'John'
character_2 = 'Luke'
print(character_1 + ': Hi ' + character_2 + ', long time no see.')
By using variables like character_1
and character_2
, you only need to change their values in one place, making the code more flexible.
String Concatenation Example:
Combining strings in Python is called concatenation. Here’s an example:
print('one' + 'twenty' + 'one') # Outputs: onetwentyone
Concatenating strings is a powerful way to build dynamic output, especially when working with variables.
The Input Function
n Python, the input
function is a fundamental tool for interacting with users. It allows your program to accept input from the user, which can then be used throughout your code. Here’s how it works:
To get user input, you call the input
function with a prompt string, like this:
address = input('Type an address: ')
This line displays the prompt 'Type an address: '
to the user and waits for them to enter a value. Once the user presses ENTER (RETURN), the input is stored in the address
variable. You can then use this variable in your program, for example:
print('This is your input address:', address)
You can also use input
to prompt users for different types of information, like names:
character_1 = input('Type the name for character 1: ')
character_2 = input('Type the name for character 2: ')
print(character_1 + ': Hi ' + character_2 + ', long time since we talked last.')
print(character_2 + ': Hi ' + character_1 + '! How have you been?')
print(character_1 + ': I have been well. Do you wanna go get lunch?')
print(character_2 + ': Yes!')
print(character_1 + ': Great, we can meet at In-n-Out burger next week')
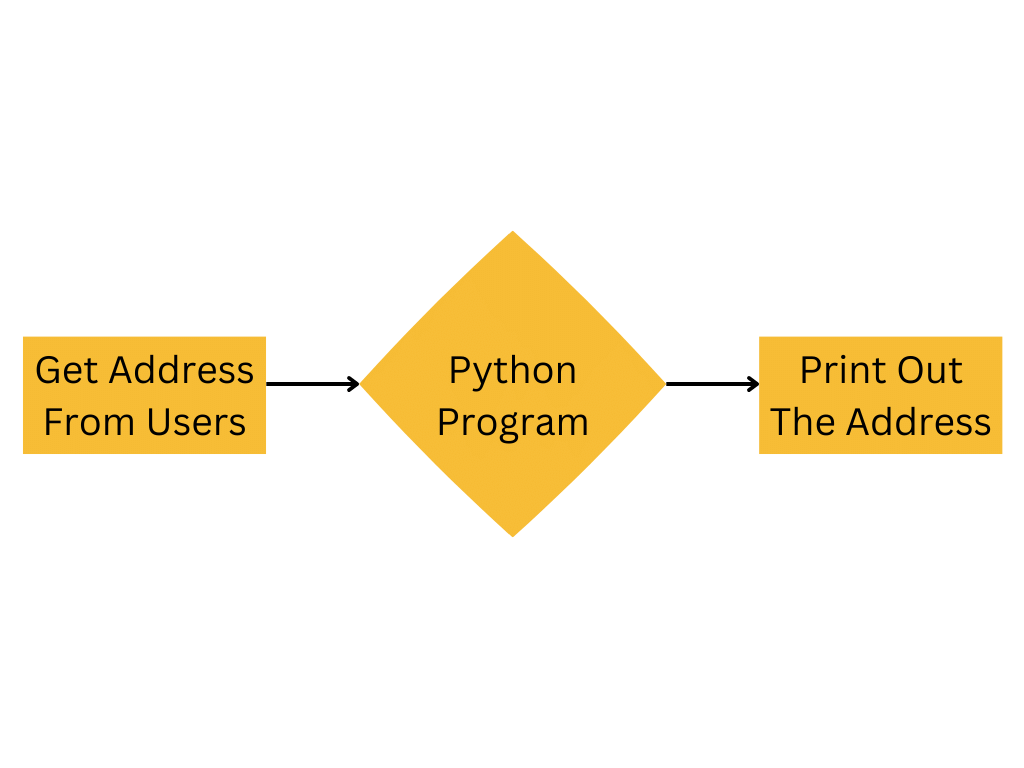
Integers
While strings are great for text, some types of data, like ages and quantities, are better represented as integers. An integer is a whole number without decimals. In Python, you can declare integers as follows:
age = 50
height = 72
When printing out these integers alongside strings, you need to convert them to strings using the str()
function:
name = 'Pierre'
email = '[email protected]'
print('His name is ' + name)
print(name + ' can be contacted at ' + email)
print('He is ' + str(age) + ' years old and is ' + str(height) + ' inches tall')
String Multiplication
String multiplication allows you to repeat a string a specified number of times. This can be particularly useful for creating visual representations, like bar charts. For example:
revenue = int(input('Business revenue: '))
cost = int(input('Business costs: '))
profit = revenue - cost
print('The business profit is: ' + str(profit))
cost_bar = '#' * int((cost / revenue) * 25)
profit_bar = '#' * int((profit / revenue) * 25)
print(' cost: ' + cost_bar)
print('profit: ' + profit_bar)
This example scales the bar charts to a maximum of 25 characters.
String Indexing
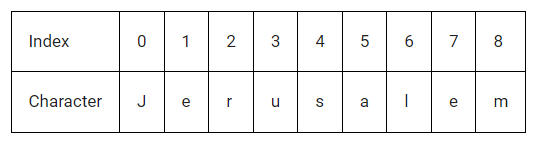
Strings in Python can be accessed by their index, which starts at 0. For example:
city = 'Jerusalem'
letter = city[4]
print(letter) # Prints 's'
Negative Indexing
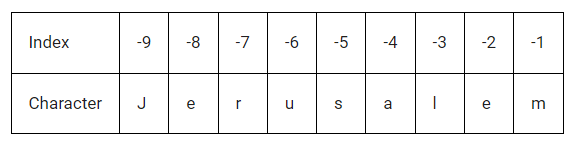
You can also use negative indexing to access characters from the end of the string:
rock = city[-9] + city[-8] + city[-1]
print(rock) # Prints 'Jem'
To get the last two characters of a string, you can use negative indexing or calculate indices with the len()
function:
Using negative indexing:
text = input('Type something: ')
print('The last two characters of your input were: ' + text[-2] + text[-1])
Using len()
:
text = input('Type something: ')
last = text[len(text) - 1]
other = text[len(text) - 2]
print('The last two characters of your input were: ' + other + last)
These features of Python string manipulation provide a powerful toolkit for handling text data in your programs.
Math
This lesson introduces some fundamental concepts of mathematical operations in Python, specifically focusing on how to use different numeric types and understand their behavior in calculations. Here’s a summary and explanation of the key points:
Basic Math Operations
Python supports several basic mathematical operations that are used to manipulate numeric values:
- Addition (+): Adds two numbers together.
- Subtraction (-): Subtracts one number from another.
- Multiplication (*): Multiplies two numbers.
- Division (/): Divides one number by another, resulting in a float.
- Exponentiation ()**: Raises one number to the power of another.
- Modulus (%): Returns the remainder of the division of one number by another.
Operator Precedence
Python follows the standard order of operations (PEMDAS/BODMAS) for evaluating expressions:
- Parentheses: Operations within parentheses are performed first.
- Exponents: Exponential operations are performed next.
- Multiplication, Division, and Modulus: These operations are evaluated from left to right.
- Addition and Subtraction: These are the last operations performed, also from left to right.
Example Analysis
For the expression x = 100 + 20 * 2
, Python evaluates multiplication before addition:
- First,
20 * 2
is computed, which equals40
. - Then,
100 + 40
is computed, resulting in140
.
For y = 200 - 100 / 2
:
- First,
100 / 2
is computed, which equals50.0
. - Then,
200 - 50.0
is computed, resulting in150.0
.
Float vs. Int
Python has two primary numeric types:
- Integer (
int
): Whole numbers without decimals. - Float (
float
): Numbers that include decimals.
When performing division in Python, even if both numbers are integers, the result will be a float. For example:
x = 100 / 2
print(type(x)) # <class 'float'>
Here, x
will be a float because division always returns a float.
Type Conversion
You can convert between integers and floats as needed. For instance, if you need to perform calculations where floating-point precision is important, you can ensure that at least one operand is a float:
x = 100 / 3 # Results in 33.333333333333336
Conversely, if you want to ensure integer division, you can use the //
operator:
x = 100 // 3 # Results in 33
Understanding these concepts is crucial for effective programming in Python, especially when dealing with mathematical computations and ensuring that operations produce the expected results.
Booleans and Boolean Expressions
In Python, booleans represent truth values: either True
or False
. This data type is fundamental for decision-making in programs, as it helps determine which code to execute based on conditions.
Boolean Values
- True: Represents a true condition.
- False: Represents a false condition.
For example:
the_sky_is_blue = True
food_is_bad = False
print(the_sky_is_blue, food_is_bad) # Output: True False
Boolean Expressions
Boolean expressions yield True
or False
values based on the conditions they evaluate. These expressions use comparison operators to compare values:
A < B
:True
ifA
is less thanB
; otherwise,False
.A <= B
:True
ifA
is less than or equal toB
; otherwise,False
.A > B
:True
ifA
is greater thanB
; otherwise,False
.A >= B
:True
ifA
is greater than or equal toB
; otherwise,False
.A == B
:True
ifA
is equal toB
; otherwise,False
.A != B
:True
ifA
is not equal toB
; otherwise,False
.
Examples of Boolean Expressions
enough_money = 1000 > 100 # True, because 1000 is greater than 100
do_the_names_match = 'Ryan' == 'Rich' # False, because 'Ryan' is not equal to 'Rich'
Using Boolean Expressions in Conditional Statements
Boolean expressions are often used in if
statements to control the flow of a program based on conditions. For example:
Checking Affordability for a Down Payment
home_cost = int(input('How much does the home cost? '))
available_funds = int(input('How much money do you have immediately available? '))
down_payment = home_cost * 0.2
if available_funds >= down_payment:
print('You can afford the down payment!')
Checking for a Specific Name
name = input('What is your name? ')
if name == 'Bob':
print('Great! Your name is Bob!')
Comparing Strings
You can also compare strings using comparison operators, though this might be less intuitive. Python compares strings lexicographically (based on alphabetical order). For instance:
print('apple' < 'banana') # True, because 'apple' comes before 'banana' alphabetically
print('apple' > 'banana') # False
In summary, booleans and boolean expressions are crucial for making decisions in your code. They help evaluate conditions and execute code based on whether those conditions are met. Understanding how to work with these expressions will allow you to write more dynamic and responsive programs.